Creating Queries from Selections¶
The FieldSelection
is used to construct a query for selecting fields from the
FieldsDatabase
. The simplest query is to gather all the fields from the database.
from lsst.sims.survey.fields import FieldSelection
fs = FieldSelection()
query = fs.get_all_fields()
print(query)
select * from Field;
The simple declination band example shown in the Getting Started section gives a good feel for how to start to use the API. Other simple band type cuts can be made from other coordinates. The strings avialable to pass to the FieldSelection.select_region()
method are:
* RA (right-ascention)
* Dec (declination)
* EL (ecliptic longitude)
* EB (ecliptic latitude)
* GL (galactic longitude)
* GB (galactic latitude)
More complicated selections can be constructed in a similar manner. We will now look at two examples from the LSST science proposals sky coverage. This is graphically shown in the plot below.
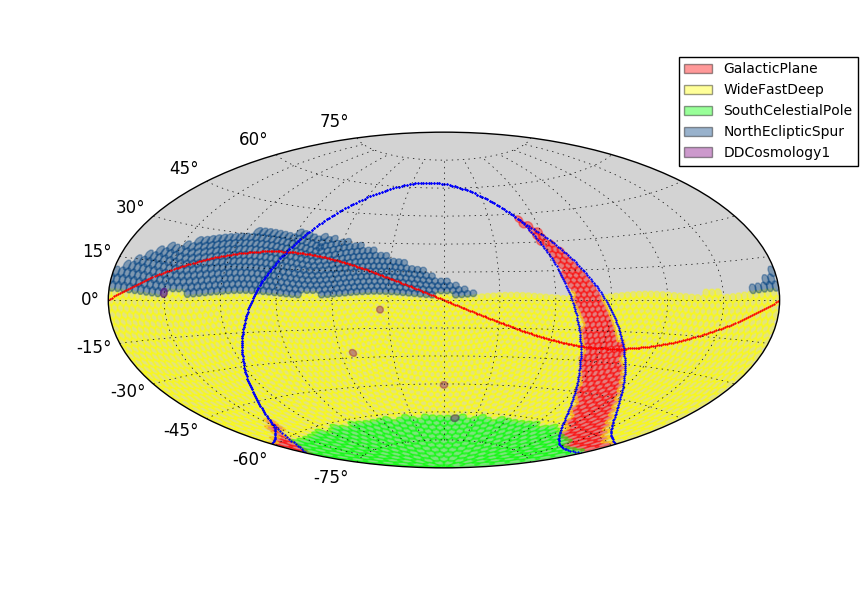
Wide, Fast, Deep Coverage¶
The Wide, Fast, Deep (WFD) proposal covers a region of the sky extending from a declination of -62.5 degrees up to 2.8 degrees. It also contains an exclusion zone around the galactic plane. The selection is created as follows.
from lsst.sims.survey.fields import FieldSelection
fs = FieldSelection()
query1 = fs.select_region("Dec", -62.5, 2.8)
query2 = fs.galactic_region(10.0, 0.0, 90.0, exclusion=True)
query = fs.combine_queries(query1, query2, combiners=('and', ))
The FieldSelection.select_region()
was shown before. The FieldSelection.galactic_region()
is used to create the region in the galactic plane. The keyword exclusion
is set to True
so that it will remove fields from any selction that it overlaps. The
FieldSelection.combine_queries()
is used to join the queries into a single call. The combiners
keyword is how this is done. This is a set of logical operations for the query joins. The size of the required tuple is one less than the number of queries to combine. A single query does not require the use of combiners
as seen before. Two queries requires the tuple to be formatted like the one shown in this example.
The parameters used in FieldSelection.galactic_region()
except exclusion
are explained in the figure below.
Galactic Plane Region Description¶
The galactic plane region is determined by an envelope as shown in the following graphic.
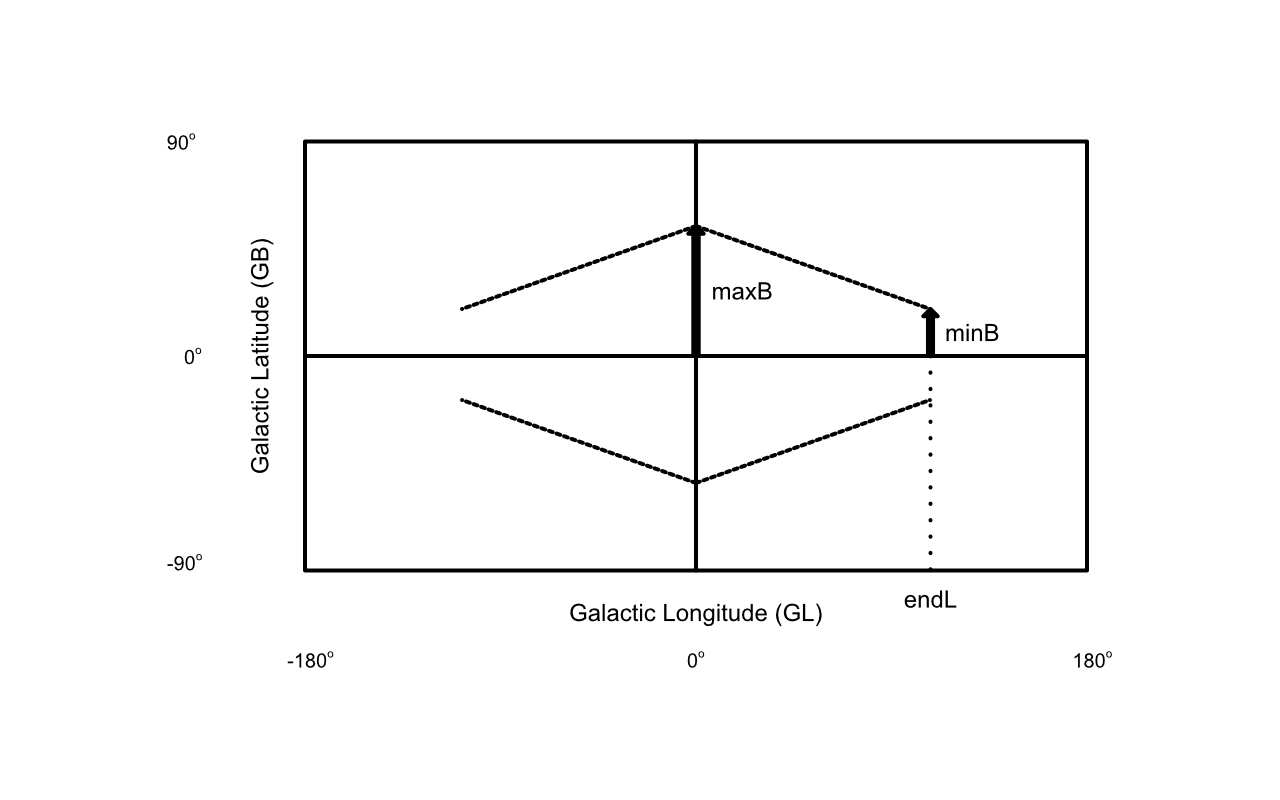
It is specified by three values:
maxB
This is the value of the maximum extent of the envelope in terms of galactic latitude (degrees) at the galactic longitude of zero degrees.
minB
This is the value of the minimum extent of the envelope in terms of galatic latitdue (degrees) at the galatic longitude specified by endL
endL
This is the end of the galactic plane envelope in terms of galactic longitude (degrees).
North Ecliptic Spur¶
The North Ecliptic Spur (NES) proposal covers a region around the ecliptic and north of 2.8 degrees declination. There is no exclusion zone for the NES. Further examples will assume a FieldSelection
instance is available as fs
. To create the NES region, the cuts are arranged as follows.
query1 = fs.select_region("EB", -30.0, 10.0)
query2 = fs.select_region("Dec", 2.8, 90.0)
query = fs.combine_queries(query1, query2, combiners=('and', ))
Selecting by Individual Fields¶
FieldSelection
has a method by which one can specify a list of field Id’s to retrieve from the field database. The LSST Deep Drilling Cosmology1 (DD1) proposal is handled via this mechanism. To duplicate the list of fields for that proposal, use the following construct.
query1 = fs.select_user_regions([290, 744, 1427, 2412, 2786])
query1 = fs.combine_queries(query1)
While this method could be used to retrieve all fields in the database, it is best to limit its use to about a dozen fields.